Password management in VCF with Powershell and PowerVCF
SDDC Manager is the core component of VMware Cloud Foundation (VCF) to manage full life cycle management tasks such as deployment, configuration, patching and upgrades. SDDC Manager can even be used to update and (automatically) rotate the passwords of all components. This feature called is Password management and can be found under Administration, Security. With Password Management it is possible to:
- Rotate All; Rotates passwords for all the accounts of the selected entity type with an auto generated password (for example all ESXi, vcenter, or NSX-T passwords)
- Rotate; Rotate a single, or all selected passwords with an auto generated password.
- Schedule Rotation; Setup a Schedule to rotate selected password.
- Update; Updates a single selected password to be updated with a manually entered password
- Remediate – If you have changed a password outside of SDDC Manager, this is the place to fix it.
One interesting thing to note is that the Schedule Rotation option is not available for ESXi related passwords.
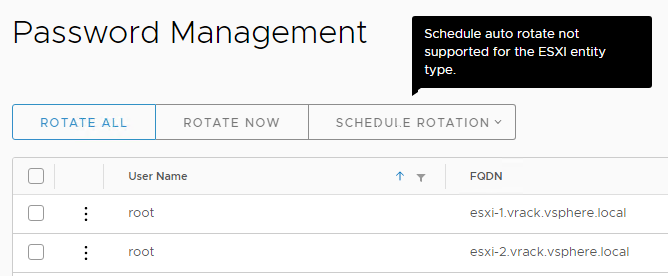
For now, you must manually rotate ESXi related passwords, which of course isn’t that hard from the UI. Although not a best practice, but a question often heard, is “how can I update the root password with a manually selected password on all ESXi hosts within a cluster”? From the UI, the only way to do this, is to click on each host, click update and change the password. Wouldn’t it be cool to do some automation with Powershell?
Before we get to the Powershell part, let’s first have a look at the VCF API. The documentation can be found online here; https://code.vmware.com/apis/1126/vmware-cloud-foundation, but you can also have a look at the API directly from the Developer Center in SDDC Manager. Here you can find the VCF API documentation, including cURL and HTTP request samples along with the responses. Beside the documentation you can use the API Explorer to run API’s directly against you live environment. This does come with a big red warning sign; Please execute with caution!
Below are all the APIs for managing Credentials:
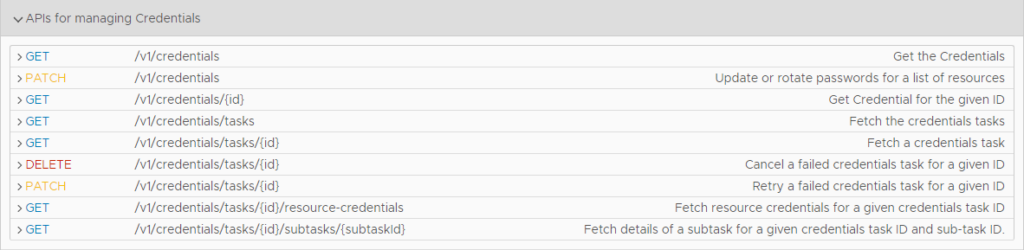
The first API is GET /v1/credentials. If you click on GET, a page expands and gives you an overview of all parameter. As you can see, you can use this api to query by resourceName, resourceIP, resourceType, domainName and AccountType.
Let’s query by Resource Type ESXI to see what the response looks like.
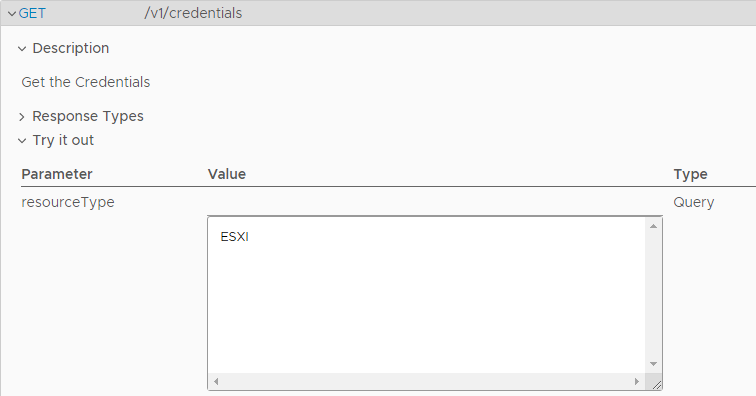
This will return a “Page of Credentials”. You can click on each returned credentials to see the values. Beside the information about the root accounts, you can also see the ESXi VCF Service accounts. If you want to filter this out even more, you can supply multiple parameters in your API request. For example; for resourceType enter value ESXI and for accountType enter value USER. This will only return the ESXi root passwords.
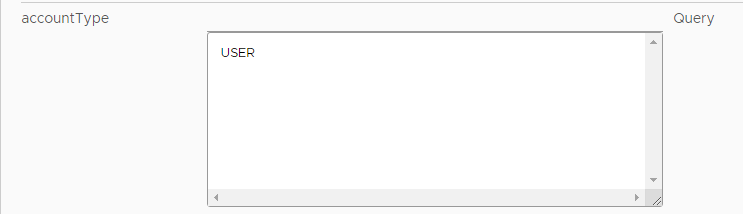
The next step is to have a look at how to Update or rotate a password. This is the second API listed, PATCH /v1/credentials API.
Click on the CredentialsUpdateSpec in the Description Field to get an example of how your (json) value could look like. You can also click on each of the elements to get more information. Initially it wasn’t always easy to find the correct and possible values from here, so I had to fall back on the API documentation a couple of times.
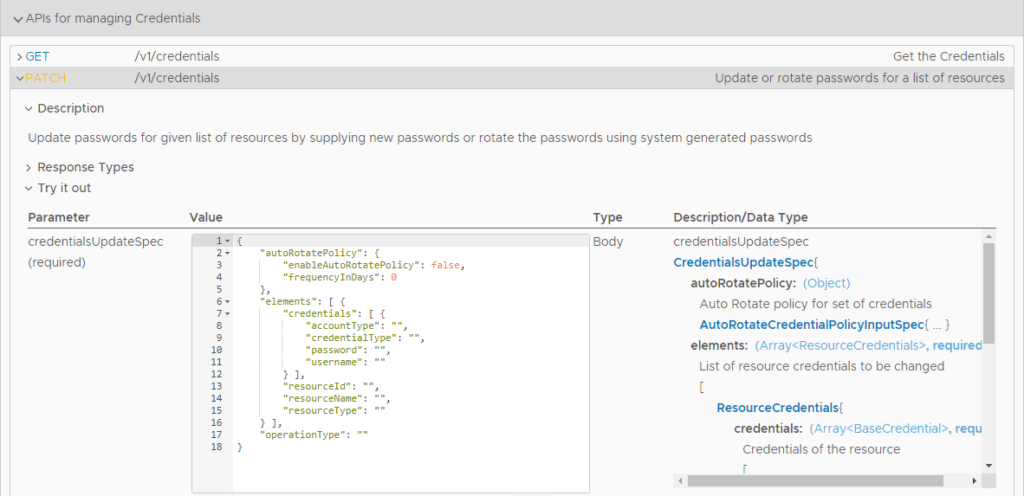
To update the root password for a host, I came up with the following json content:
{
"elements": [ {
"credentials": [ {
"credentialType": "SSH",
"username": "root",
"password": "VTAMblogFTW!9751"
} ],
"resourceName": "esxi-1.vrack.vsphere.local",
"resourceType": "ESXI"
} ],
"operationType": "UPDATE"
}
Click Execute and watch the task progress. If the task was successful, you can check the new password with the first API call. (Hint; query by resourceName).
Now we know how to change passwords via the API (Explorer), let’s see if we can make life easier by doing some automation with PowerShell. The good news is that there is a PowerShell module written for VCF called PowerVCF. This module takes away a lot of PowerShell REST scripting by providing a great number of cmdlets. Note that this is not an officially supported VMware PowerShell Module, but created by some smart people from VMware and Dell.
You can find the PowerVCF module on github https://github.com/PowerVCF/PowerVCF
and in the PowershellGallery https://www.powershellgallery.com/packages/PowerVCF/2.1.3
For this blogpost, I used the following
- VCF 4.3 Test environment
- PowerShell 7.1.2 (installed on SDDC Manager)
- PowerVCF 2.1.3 module
- MS Visual Studio Code
I have created 2 separate scripts; one script to update- and one script to rotate the passwords on all selected hosts:
- “VCF-RotateESXiRootPwd.ps1”
- “VCF-UpdateESXiRootPwd.ps1”
You can find the scripts on my [github] page.
There are a couple of variables in the scripts that need your attention:
$jsonFilePath; depending on if you run the script from SDDC Manager or from a Windows System, this variable needs to be set differently. This path variable is used to create the json files needed to update/rotate the password. The cmdlet to change the password (set-vcfcredential) only accepts a JSON file for input. Note that the created JSON files will not be removed after you run the script.
#Configure Path to store json configuration files
#$jsonFilePath = "C:\Temp\VCF\" #Windows System
$jsonFilePath = "/root/" #Linux System
$VCFClusterName and/or $vcfHosts. Here you can select on which host(s) to update/rotate the password
#Select All Hosts from Given Cluster
$VCFClusterName = "SDDC-Cluster1"
$VCFClusterId = (Get-VCFCluster -name $VCFClusterName).id
$vcfHosts = Get-VCFHost |Where-Object {$_.cluster.id -eq $VCFClusterId} |Select-Object fqdn, id |Sort-Object fqdn
# Or select ALL hosts from VCF | You may want to filter here
#$vcfHosts = Get-VCFHost |Select-Object fqdn
I choose to run the script directly from the SDDC Manager:
- SSH to the SDDC Manager and login with the vcf account
- su – to root
- pwsh command to start PowerShell
login as: vcf
Welcome. This system is for authorized users only.
vcf@10.0.0.4's password:
Last login: Wed Aug 25 18:27:52 2021 from 10.0.0.253
vcf@sddc-manager [ ~ ]$ su -
Password:
root@sddc-manager [ ~ ]# pwsh
PowerShell 7.1.2
Copyright (c) Microsoft Corporation.
https://aka.ms/powershell
Type 'help' to get help.
PS /root>
The installation and import of the PowerVCF module is included in the script, but you can run a manual install and import of the PowerVCF module:
PS /root> install-module PowerVCF
PS /root> import-module PowerVCF
PS /root> Get-Module PowerVCF |Select Name, Version
Name Version
---- -------
PowerVCF 2.1.3
Copy the scripts over to the SDDC Manager (or create new files and paste the contents) and run the script;
PS /root> vi VCF-UpdateESXiRootPwd.ps1
PS /root> ./VCF-UpdateESXiRootPwd.ps1
Successfully Requested New API Token From SDDC Manager: sddc-manager.vrack.vsphere.local
Current root credentials for VCF Host esxi-1.vrack.vsphere.local : VTAMblogFTW!9751
Current root credentials for VCF Host esxi-2.vrack.vsphere.local : VTAMblogFTW!9751
Current root credentials for VCF Host esxi-3.vrack.vsphere.local : VTAMblogFTW!9751
Current root credentials for VCF Host esxi-4.vrack.vsphere.local : VTAMblogFTW!9751
id status
-- ------
a17aeb35-6915-48b2-bac9-2955a98d7624 IN_PROGRESS
8fa19868-bf14-44c9-a315-3e7c8e965b7c IN_PROGRESS
44f5feb3-231f-4aab-a5f5-233c26c3160d IN_PROGRESS
82a17905-2b12-40d2-aa5e-fd70606c519a IN_PROGRESS
Current root credentials for VCF Host esxi-1.vrack.vsphere.local : YourNewVMwPwd!1357
Current root credentials for VCF Host esxi-2.vrack.vsphere.local : YourNewVMwPwd!1357
Current root credentials for VCF Host esxi-3.vrack.vsphere.local : YourNewVMwPwd!1357
Current root credentials for VCF Host esxi-4.vrack.vsphere.local : YourNewVMwPwd!1357
The rotate password script works the same and shows the same sort of output:
PS /root> vi ./VCF-RotateESXiRootPwd.ps1
PS /root> ./VCF-RotateESXiRootPwd.ps1
Successfully Requested New API Token From SDDC Manager: sddc-manager.vrack.vsphere.local
Current root credentials for VCF Host esxi-1.vrack.vsphere.local : YourNewVMwPwd!1357
Current root credentials for VCF Host esxi-2.vrack.vsphere.local : YourNewVMwPwd!1357
Current root credentials for VCF Host esxi-3.vrack.vsphere.local : YourNewVMwPwd!1357
Current root credentials for VCF Host esxi-4.vrack.vsphere.local : YourNewVMwPwd!1357
id status
-- ------
60ee717c-ed22-4909-a7e3-521b79b8558e IN_PROGRESS
582197b9-10c2-4a87-8969-ff69d70c335b IN_PROGRESS
18291e03-65f6-4b5b-b0d6-5f005e693d8b IN_PROGRESS
55dd0a6c-1182-4da4-a073-48757016cd32 IN_PROGRESS
Current root credentials for VCF Host esxi-1.vrack.vsphere.local : r@Q3#7pvf*9$XKaU^2Z6
Current root credentials for VCF Host esxi-2.vrack.vsphere.local : zR*07ND^Y$3y@8ajo#bh
Current root credentials for VCF Host esxi-3.vrack.vsphere.local : v0^R#94iI5X8@1Ms27yB
Current root credentials for VCF Host esxi-4.vrack.vsphere.local : g9U*GBH2#O1PS87u6el0
That’s it. There are lots of other useful cmdlets in PowerVCF to explore. You can list all available commands in the module with: get-command -Module PowerVCF