Generate an Access- and Bearer Token for Aria Automation API Authentication
For automating things in Aria Automation, my preferred method is PowerShell calling the API’s.
I’ve been using this method for quite some time as you may have seen in my previous blogposts. Although VMware KB89129 describes the process to retrieve the Access Token, I noticed some people are still struggling to get started, hence I’ll share and explain this specific piece of code.
The first part of the script defines the variables:
# vRA VARIABLES
$vraName = "vra"
$domain = "infrajedi.local"
$vraHostname = $vraname+"."+$domain
$vraUsername = "configadmin"
$vraPassword = "VMware1!" #note use ` as escape character for special chars like $
$vraUserDomain = "System Domain" #Use "System Domain" for local users", otherwise use the AD domain.
- Note: The
$vraHostname
variable. You may also use static values here. In my example the$vraHostname
variable will result in vra.infrajedi.local and will be used later to connect. - Note: the
$vraUserDomain
variable and the comments I placed behind it.
The second part generates the initial header we need to make a REST call:
# Create Header
$header = New-Object "System.Collections.Generic.Dictionary[[String],[String]]"
$header.Add("Accept", 'application/json')
$header.Add("Content-Type", 'application/json')
The third part generates the Refresh Token by calling out the Identity Service API:
# Generate Refresh Token
$uri = "https://$vraHostname/csp/gateway/am/api/login?access_token" #Identity Service API
$data=@"
{
"username" : "$vraUsername",
"password" : "$vraPassword",
"domain" : "$vraUserDomain"
}
"@
try {
$response = Invoke-RestMethod -Method Post -Uri $uri -Headers $header -Body $data
} catch {
write-host "Failed to Connect to host: $vraHostname" -ForegroundColor red
Write-Host "StatusCode:" $_.Exception.Response.StatusCode.value__
Write-Host "StatusDescription:" $_.Exception.Response.StatusDescription
break
}
- Note: For PowerShell JSON data, I prefer to use plain JSON. For PowerShell you have to pay special attention to the
@”
opening and“@
closing, as well as the correct use of curly brackets{}
. - A great way to validate and correct your JSON data is the [jsonlint] website (beware you don’t copy passwords, just in case…)
The output of $response
is:
refresh_token
-------------
s9qZqQ6BhKKU0lESywT6Af3ledtmHRaE
Since we only need the value for the refresh token, a new variable $vraRefreshToken
is created:
$vraRefreshToken = $response.refresh_token #vraRefreshtoken used to obtain Access (Bearer) token.
The $vraRefreshToken
will be used in the body of the REST call to the IAAS API”
# Generate Access Token
$data =@"
{
"refreshToken" : $vraRefreshToken
}
"@
$uri = "https://$vraHostname/iaas/api/login"
try {
$response = Invoke-RestMethod -Method Post -Uri $uri -Headers $header -Body $data
} catch {
write-host "Failed to Connect to host: $vraHostname" -ForegroundColor red
Write-Host "StatusCode:" $_.Exception.Response.StatusCode.value__
Write-Host "StatusDescription:" $_.Exception.Response.StatusDescription
break
}
The (shortened) output of $response
looks like this:
tokenType token
--------- -----
Bearer eyJ0eXAiOiJKV1QiLCJhbydml......jZS5wCJpYXQiOjE3MDAxNTAyNjEsI
Finally I have created a $vraBearerToken
variable containing the value of the token and added an “Authorization” entry to the existing header:
$vraBearerToken = $response.token
#Add Bearer Authentication to Header
$header.add("Authorization", "Bearer $vraBearerToken")
From here you can just include the header in any follow up API call. For example retrieving the Cloud Accounts:
#Get vSphere Cloud Accounts
$uri = "https://$vraHostname/iaas/api/cloud-accounts-vsphere"
$response = Invoke-RestMethod -Method Get -Uri $uri -Headers $header
- -> Examine the $response variable
- -> Examine $response.content to lookup the id for the Cloud Account (for example)
Have a look at my github page for additional examples.
Bonus add-on:
The $vraBearerToken
can also be used directly in the Swagger UI which can be found here https://<vrahostname>/automation-ui/api-docs/
Click on any of the
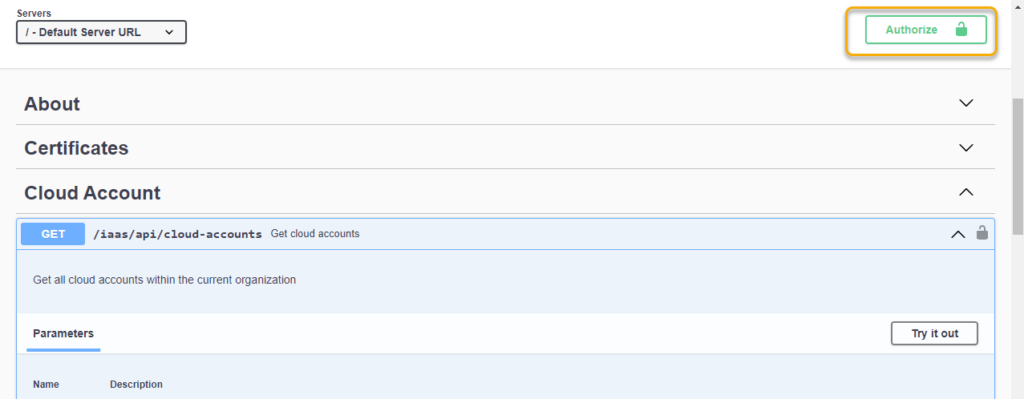
Click Authorize, Type Bearer followed by the $vraBearerToken
value (just like it is in the header from the PowerShell script), Click Authorize and Close. Note that you will not get an error message if the token is incorrect (until you run an api call from the Swagger UI resulting in 401)
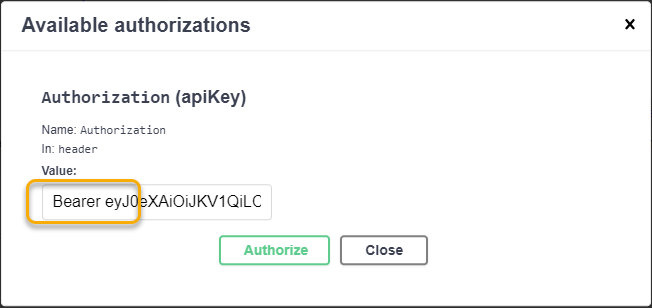
Now you can open any API call, click “Try it out” and clik “Execute” to run your request. Depending on the request you may have to enter values for required parameters.