Create a user in the VIDM System Directory
While running into some issues with Aria Automation I needed to create a new testuser. Since it was not possible at that time to create an Active Directory account, I decided to create a local account in the VIDM System Directory.
Sidenote: depending on where you are in the UI or documentation the product may be called VIDM, VMware Identity Manager or Workspace One Access. I’ll refer to VIDM.
Setup / Configuration
During testing my setup / configuration was as follows:
- vSphere 8.0U2b
- Visual Studio Code 1.87.2
- Powershell 7.4.1
- Aria Suite Lifecycle 8.16.0.4 (23334789)
- VIDM 3.3.7, with HW-189454-3.3.7.0 hotfix applied.
- No multi-tenancy setup
- Aria Automation 8.16.0
Create a user through the UI
To create a user in the VIDM System Directory:
- Login to VIDM with the admin account
- Open the Administration Console from the top right
- Select the tab “Users & Groups”
- Click “Add User”
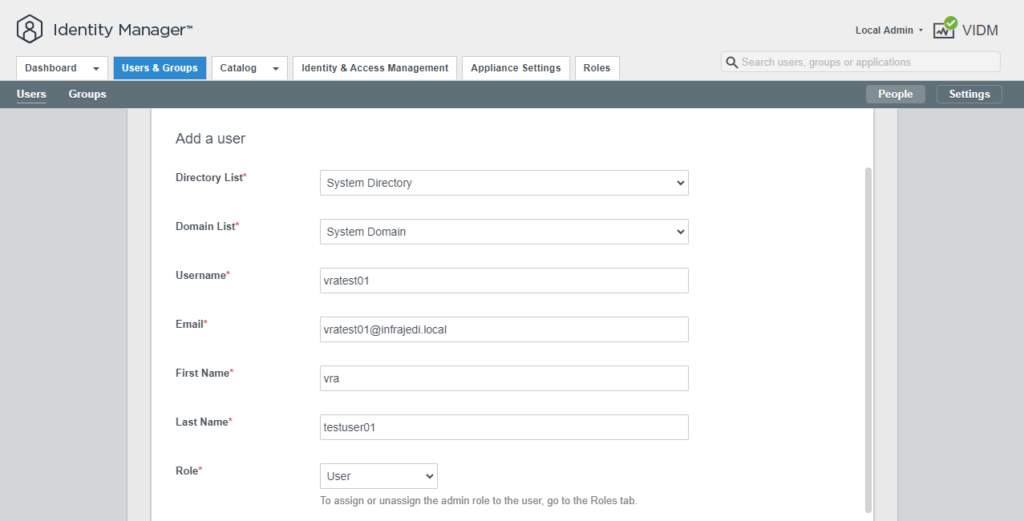
After you click Add, an error message showed up “user has been added as a user, but an activation email could not be sent to <email address>. Check the SMTP configuration is correct.“
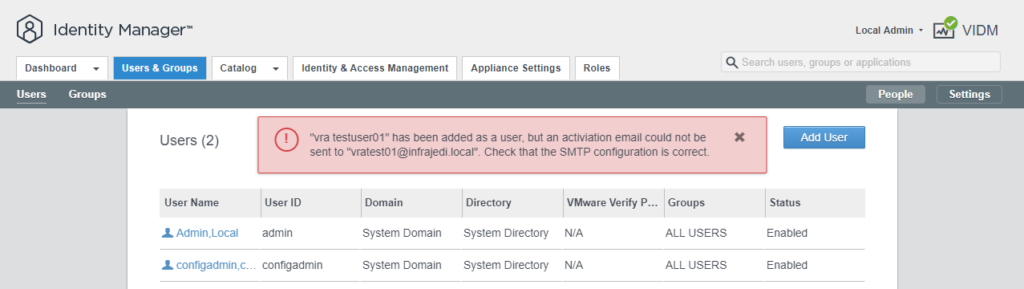
Besides the typo “activiation” in the error message; I have created a user, but since I do not have SMTP setup, the password is unknown.
So much for the UI. Luckily there is an API way to update a user’s password and/or create a new user with password with the API. There is no built-in Swagger interface for VIDM; the documentation can be found [here].
Since PowerShell is my scripting tool of choice, I decide to poke around in the API and create some scripting to create a user, set a password and delete a user
Request (oAuth) SessionToken through Webbrowser
The first thing you need to do, is to request a (oAuth) SessionToken. You can grab the SessionToken with the help from the developer tools option from your webbrowser after logging in to VIDM.
- Start Google Chrome
- Login to VIDM with the admin account on the System Domain
- Activate Develeper Tools by Clicking on the top right-hand menu icon with the 3 dots.
- Select More Tools, Developer Tools
- In the Developer Tools Screen Select Application and look for the Cookies section.
- In there pick the VIDM URL to reveal the HZN SessionToken
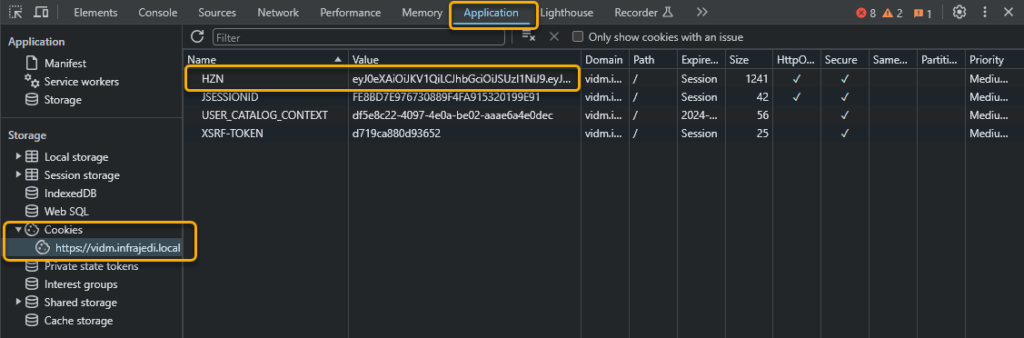
Copy the HZN sessionToken value to use it in any scripting tool you prefer. However, it would be even better to script this part as well! Let see how this works in the next section.
Request (oAuth) SessionToken with API through Powershell
The uri to request an (oAuth) SessionToken is: https://{vidmHostname}/SAAS/API/1.0/REST/auth/system/login
For the header we only need to configure two key/value parameters:
- Accept : ‘application/json’
- Content-Type: ‘application/json’
For the body of the request, we need username, password and issueToken set to true:
#VARIABLES
$vidmHostname = "vidm.infrajedi.local"
$vidmAdminUsername = "admin"
$vidmAdminPassword = "VMware01!"
#Create Initial Header to request Session Token
$header = New-Object "System.Collections.Generic.Dictionary[[String],[String]]"
$header.Add("Accept", 'application/json')
$header.Add("Content-Type", 'application/json')
$authBody = @"
{
"username": "$vidmAdminUsername",
"password": "$vidmAdminPassword",
"issueToken": "true"
}
"@
#Request Session Token
$uri = "https://$vidmHostname/SAAS/API/1.0/REST/auth/system/login"
$authResponse = Invoke-RestMethod -Uri $uri -Headers $header -Method Post -Body $authBody
$vidmSessionToken = $authResponse.sessionToken
With the SessionToken retrieved you can now add this to the header:
#Add SessionToken to Header
$header.add("Authorization", "HZN $vidmSessionToken")
Create VIDM user with password through API
Note: before you really start adding/deleting/breaking things create a snapshot and/or backup and make sure to test in dev/test before production! There is no error/fault handling, just straight forward (lazy) scripting
With the SessionToken retrieved and the updated header you can now go ahead and run your API requests. In this example I will create a user with a configured password in the VIDM System Domain.
The uri to create a user is: https://<vidmHostname>/SAAS/jersey/manager/api/scim/Users
In the most simple form the body contains the name, givenName, familyName, userName, password and emails. Note that if you have setup multi-tenancy you may need to add additional fields. See the below example to create a new user “vratest01”:
#####################################################
# Create New User with password in System Directory #
#####################################################
$newVidmUser = "vratest01"
$newVidmPassword = "VMware01!"
$newVidmEmail = "vratest01@infrajedi.local"
$body = @"
{
"name": {
"givenName": "vra",
"familyName": "test"
},
"userName": "$newVidmUser",
"password": "$newVidmPassword",
"emails": [
{
"value": "$newVidmEmail"
}
]
}
"@
$uri = "https://$vidmhostname/SAAS/jersey/manager/api/scim/Users"
$createUserResponse = Invoke-RestMethod -Uri $uri -Headers $header -Method Post -Body $body
$vidmUserId = $createUserResponse.id
(Re)Set VIDM user password through API
To reset a user’s password, you will first have to retrieve the userId. After that it’s a simple Patch call to https://<vidmHostname>/SAAS/jersey/manager/api/scim/Users/<userId> with a body containing the password
##########################
# Reset Password of user #
##########################
#First Get specific User
$vidmUsername = "vratest01"
$uri = "https://$vidmhostname/SAAS/jersey/manager/api/scim/Users?filter=username%20eq%20%22$vidmusername%22"
$userResponse = Invoke-RestMethod -Uri $uri -Headers $header -Method Get
$vidmUserId = $userResponse.Resources.id
$vidmUserId
#Set Password
$uri = "https://$vidmhostname/SAAS/jersey/manager/api/scim/Users/"+$vidmUserId
$body = @'
{
"password": "VMware01!"
}
'@
$resetUserPassword = Invoke-RestMethod -Uri $uri -Headers $header -Method patch -Body $body
Delete VIDM user through API
To delete a user, you also will first have to retrieve the userId. After that it’s a simple Delete call to https://<vidmHostname>/SAAS/jersey/manager/api/scim/Users/<userId>
###################################
# Delete User in System Directory #
###################################
#First Get specific User
$vidmUsername = "vratest01"
$uri = "https://$vidmHostname/SAAS/jersey/manager/api/scim/Users?filter=username%20eq%20%22$vidmusername%22"
$userResponse = Invoke-RestMethod -Uri $uri -Headers $header -Method Get
$vidmUserId = $userResponse.Resources.id
$vidmUserId
#delete user
$uri = "https://$vidmHostname/SAAS/jersey/manager/api/scim/Users/"+$vidmUserId
$deleteUserResponse = Invoke-RestMethod -Uri $uri -Headers $header -Method Delete
Closing thoughts
A Powershell script “VIDM-UserManagement.ps1” with all the above can be found on my [Github] page.
As mentioned earlier before you really start adding/deleting/breaking things create a snapshot and/or backup and make sure to test in dev/test before production!
After you have created a VIDM user in the System Domain, don’t forget to set Organizational Roles and Permissions in Aria Automation